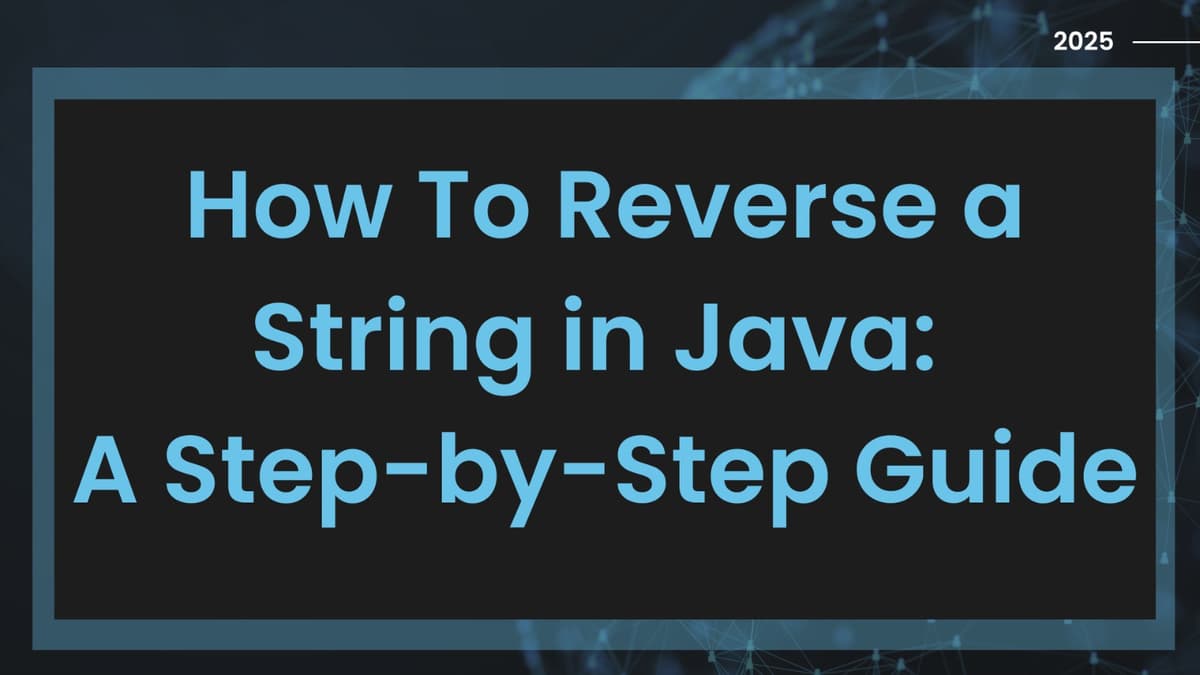
How To Reverse a String in Java: A Step-by-Step Guide
Published: 2025-01-02 12:20:51
Reversing a string is a common problem that developers face when learning programming. In Java, there are multiple ways to reverse a string, using both built-in functions and manual methods. Whether you're working with algorithms in competitive programming, tackling coding challenges, or simply enhancing your Java skills, understanding how to reverse a string is a key concept. Let’s explore how to reverse a string in Java using different approaches.
How To Reverse a String in Java: Step by Step Guide
Reversing a string can be done in several ways in Java. Below are different methods to accomplish this:
- Reverse a String in Java Using For Loop
- Reverse a String in Java Using StringBuilder
- Reverse a String in Java Without Using Inbuilt Functions
- Reverse a String in Java (LeetCode Style)
1. Reverse a String in Java Using For Loop
One of the simplest ways to reverse a string in Java is by using a for loop. The idea is to iterate through the string in reverse order and append each character to a new string. Below is the step-by-step approach:
Steps:
- Initialize an empty string to store the reversed result.
- Start a for loop, iterating through the input string from the last character to the first.
- Append each character to the new string.
- Finally, return the reversed string.
Machine Learning Career Opportunities
Mobile App Developer Career Scope in India
Mobile App Development Training and Certification
Native vs Cross-Platform Development
Next.js vs Other JavaScript Frameworks
Code Example:
public class ReverseString {
public static void main(String[] args) {
String input = "Hello, Java!";
String reversed = "";
// Loop through the string in reverse order
for (int i = input.length() - 1; i >= 0; i--) {
reversed = input.charAt(i);
}
System.out.println("Reversed String: " reversed);
}
}
This method works well for small strings, but it can be inefficient for large strings due to the immutable nature of strings in Java. Each time you append a character, a new string is created.
Also Read This: Top Reasons to Learn Redux Today
2. Reverse a String in Java Using StringBuilder
A more efficient way to reverse a string in Java is by using the StringBuilder class. This class provides a reverse() method that can reverse a string easily without the overhead of creating multiple string objects.
Steps:
- Create a StringBuilder object and initialize it with the original string.
- Use the reverse() method to reverse the string.
- Convert the StringBuilder object back to a string and print the result.
Code Example:
public class ReverseString {
public static void main(String[] args) {
String input = "Hello, Java!";
// Use StringBuilder to reverse the string
StringBuilder sb = new StringBuilder(input);
String reversed = sb.reverse().toString();
System.out.println("Reversed String: " reversed);
}
}
Also Read This: Best coding bootcamps in India
Advantages of StringBuilder:
- StringBuilder is more memory efficient than concatenating strings using the operator.
- The reverse() method is optimized and much faster than manually iterating through the string with a loop.
Programming Courses After 12th
Programming Languages for Your Career
Project Ideas for Android App Development
Top 10 Key Reasons to Learn Coding
Top 5 Programming Languages IT Professionals Should Learn in 2024
3. Reverse a String in Java Without Using Inbuilt Functions
If you want to reverse a string manually, without relying on built-in methods like reverse(), you can do so by converting the string to a character array and swapping the characters.
Steps:
- Convert the string into a character array.
- Swap characters from the start and end of the array until you reach the middle.
- Convert the character array back into a string and print the result.
Code Example:
public class ReverseString {
public static void main(String[] args) {
String input = "Hello, Java!";
// Convert the string to a character array
char[] charArray = input.toCharArray();
int start = 0;
int end = charArray.length - 1;
// Swap characters until we reach the middle
while (start < end) {
char temp = charArray[start];
charArray[start] = charArray[end];
charArray[end] = temp;
start ;
end--;
}
// Convert the character array back to a string
String reversed = new String(charArray);
System.out.println("Reversed String: " reversed);
}
}
This approach directly manipulates the array of characters, offering a hands-on way to reverse the string without relying on built-in methods. It is a good practice for understanding low-level string manipulations.
Also read This: AI and Machine Learning Courses: FAQs
4. Reverse a String in Java Leetcode
On platforms like LeetCode, reversing a string is a common interview question. The problem often comes with additional constraints, such as solving the problem without using inbuilt functions or libraries. In such cases, you can use a variety of techniques, including a recursive approach or the two-pointer technique.
LeetCode Approach:
- Initialize two pointers, one at the beginning (left) and one at the end (right) of the string.
- Swap the characters at the left and right pointers.
- Move the pointers toward each other until they meet in the middle.
Code Example:
public class ReverseString {
public static void reverse(char[] s) {
int left = 0;
int right = s.length - 1;
// Swap characters using two pointers
while (left < right) {
char temp = s[left];
s[left] = s[right];
s[right] = temp;
left ;
right--;
}
}
public static void main(String[] args) {
char[] input = {'H', 'e', 'l', 'l', 'o'};
reverse(input);
System.out.println("Reversed String: " new String(input));
}
}
In this LeetCode-style solution, the input string is treated as a character array, and the two-pointer technique is applied to reverse it. This approach is optimal and widely used in competitive programming due to its simplicity and efficiency.
To Join Our Bootcamp Register Here: https://www.codewithtls.com/admission
Reversing a string in Java can be achieved through various techniques, depending on your use case and performance requirements. Whether you prefer using a for loop, StringBuilder, or manipulating characters manually, Java offers multiple ways to accomplish this task. For more complex scenarios like LeetCode-style problems, utilizing two-pointer techniques or recursion might be required.
By understanding these methods, you not only improve your problem-solving skills but also gain a deeper insight into Java’s powerful features, like StringBuilder and character array manipulations.
Do you want to learn JAVA in just 3 months?
Join Code with TLS, the best IT course provider, and get classes at your convenience—available both online and offline.
Why Choose Code with TLS?
- Most Affordable Fees: Learn JAVA without breaking the bank.
- Job Assistance: We support you in landing your dream job.
- 100% Salary Hike: Achieve significant career growth through our course.
- Certification: Showcase your skills on LinkedIn or your resume with our recognized certification.
Start your journey toward mastering JAVA today! Visit Here: Code with TLS Course
FAQs
1. What is the easiest way to reverse a string in Java? The easiest way to reverse a string in Java is by using the StringBuilder class. It provides a built-in reverse() method which is efficient and fast.
2. Can I reverse a string in Java without using the reverse() method? Yes, you can reverse a string in Java by manually iterating through the string with a for loop or by using a character array and swapping characters.
3. How can I reverse a string for LeetCode problems in Java? For LeetCode problems, a two-pointer technique can be used, where you swap characters at the start and end of the string and move the pointers towards the center.
4. What is the time complexity of reversing a string in Java? The time complexity of reversing a string using the StringBuilder reverse method or manual iteration is O(n), where n is the length of the string.
5. Why should I use StringBuilder instead of a for loop to reverse a string? StringBuilder is more efficient than using a for loop because it avoids creating multiple immutable string objects, thus improving memory usage and performance.
6. Is reversing a string in Java a common interview question? Yes, reversing a string is a common problem in coding interviews, especially for beginner-level Java developers, as it tests your understanding of string manipulation.